How to Deploy an ERC20 Token Contract
This guide will walk you through deploying an ERC 20 token contract using the Remix IDE. You can use this ERC20 token in our Onchain reward app to incentivize your users to participate in your campaign.
What You Will Need
- A Web3 Wallet (eg. Metamask, Phantom or any Wallet Connect enabled wallet)
- Testnet tokens to deploy the contract (eg. Sepolia, Arthera, Dojima etc.)
- Access to a modern web browser (eg Brave, Chrome, Firefox)
Why an ERC20 Token Reward?
ERC20 tokens are fungible, meaning they can be exchanged on a one-to-one basis. This makes them ideal for creating rewards, loyalty points, and other fungible assets. You can consider them as digital certificates of ownership for fungible items, with each token representing a specific value.
Steps to Deploy an ERC20 Contract
Step 1: Getting Testnet Tokens
You will need testnet tokens to deploy the contract. We are using the Arthera testnet (opens in a new tab) in this guide. You can get testnet tokens from the Arthera faucet here (opens in a new tab)
If you don't have Arthera testnet enabled in your wallet, you can add it here (opens in a new tab).
Step 2: Writing and Deploying the Contract
There are many ways to deploy an ERC20 token contract. In this guide, we will use the Remix IDE, a popular online Solidity IDE.
Contract logic can vary depending on the use case. We will be using a simple ERC20 contract in this guide. To follow along copy and paste this code into the Remix IDE.
// SPDX-License-Identifier: GPL-3.0
pragma solidity 0.8.20;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
/**
* @title USDCT Token
* @author Bandit
* @dev A simple ERC20 token contract representing Test USDC (USDCT).
*/
contract XP is ERC20 {
/**
* @dev Initializes the USDCT token contract.
*/
constructor() ERC20("Test XP", "XP") {
_mint(msg.sender, 1000000); // Mint 1,000,000 tokens and assign them to the contract deployer
}
/**
* @dev Returns the number of decimals used by the USDCT token.
* @return The number of decimals.
*/
function decimals() override public pure returns(uint8) {
return 1;
}
}
- In remix, select the compiler version based on the version of the contract you are using. You can compile the contract by clicking on the "Compile" tab and then click on the "Compile XP.sol" button.
- After compiling the contract you can deploy it using Injected Provider. Make sure you are connected to the blockchain network you want to deploy the contract on. Click on the "Deploy" button to deploy the contract.
- Confirm the transaction in your wallet and wait for the contract to be deployed.
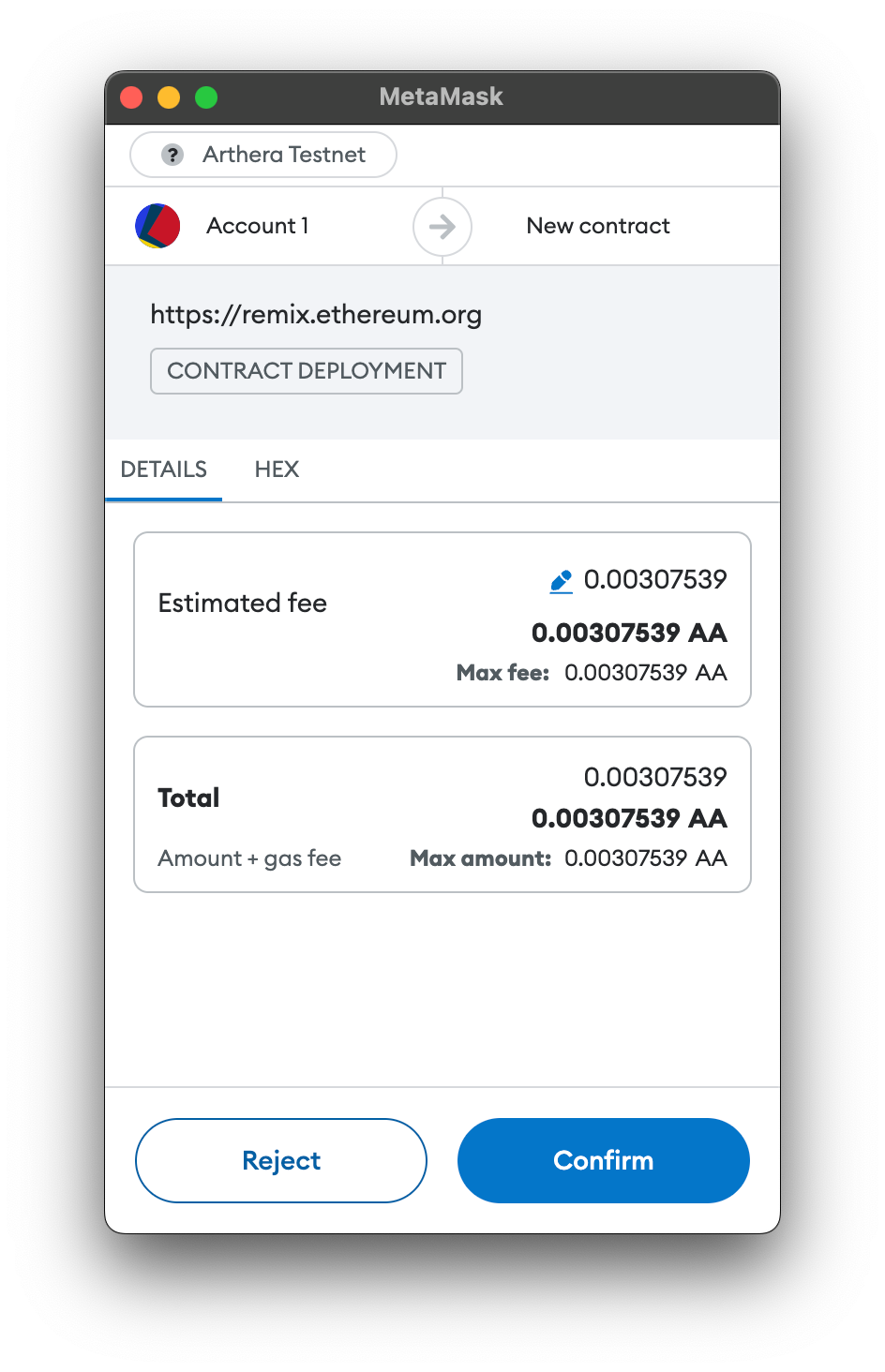
Step 3: Approve Reward Distributor Address
Once the contract is deployed, you need to approve the reward distributor address to transfer tokens. You can do this by calling the approve
function in the contract.
Reward Distributor Address: Our reward distributor address transfers the token reward you have setup in our ERC20 Reward App. It needs to approved in the token contract.
The following table shows the distributor addresses you would need to use based on the network you are deploying the contract on:
Blockchain | Reward Distributor Address |
---|---|
Holesky | 0x8e5C6ED48bB51dB26538160712321b90F535F80b |
Dojima Testnet | 0xE8D75b895bcAFDB67A6406B903dd6968dAB5d459 |
Arthera Testnet | 0x97Cbe9e8189a468DdEc65639bDA897ac7e94e8B0 |
Arthera Mainnet | 0xf7A3bD044caC063a3245332879109CE2d99E63DD |
Binance Smart Chain | 0xE22Ec9D2e3B74eEab498a46f20D352E5350bd864 |
Polygon Mainnet | 0x3329c686DF899dFC4d1Ea3e8ACcceB187b3973d0 |
- Go to the contract in Remix and click on the "Deployed Contracts" tab. Copy the reward distributor address and paste it in the approve field and set the value to the number of tokens it can transfer. Click on "transact" button to approve the distributor address.
- Confirm the spending cap in your wallet and approve the transaction.
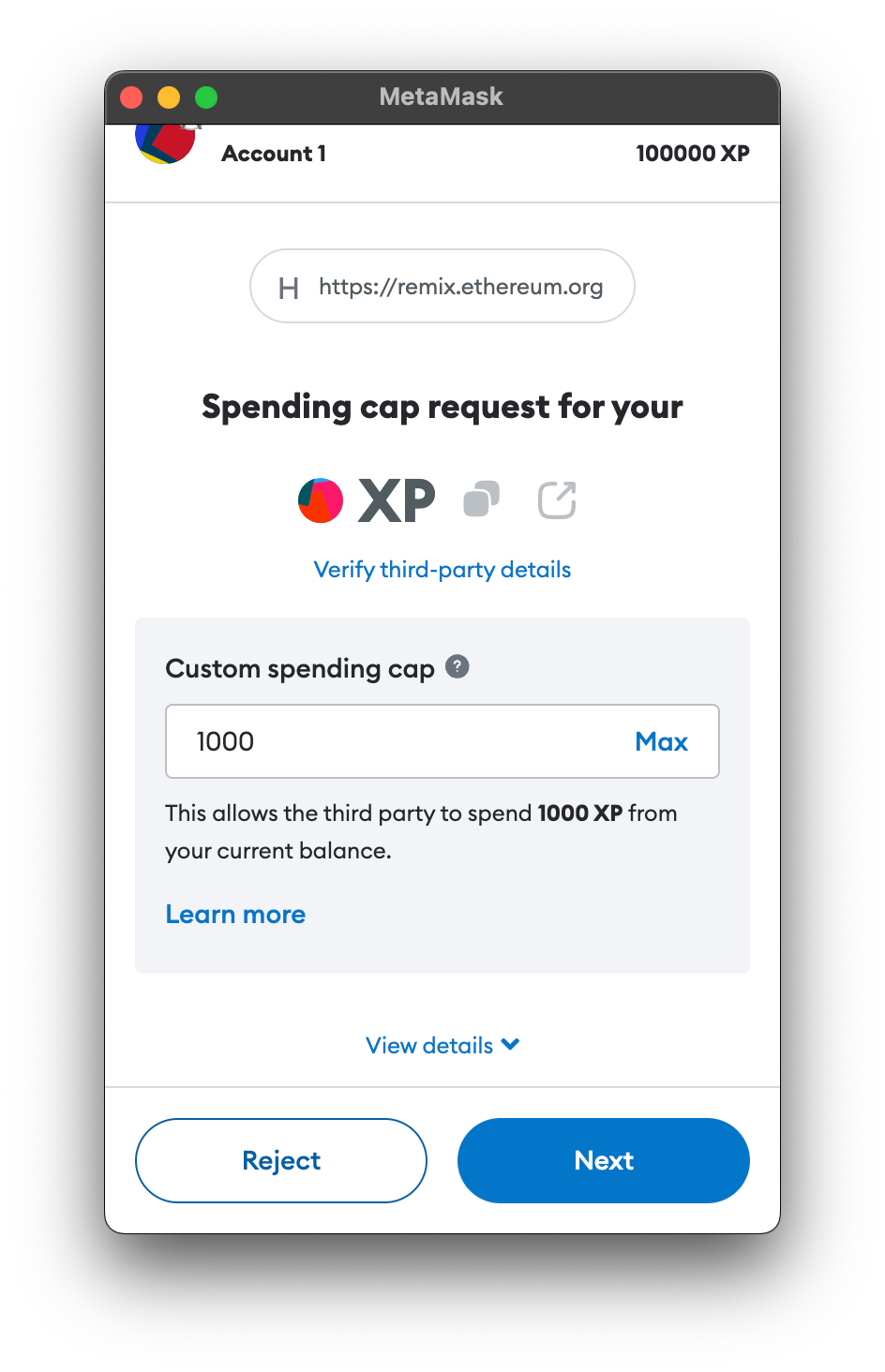
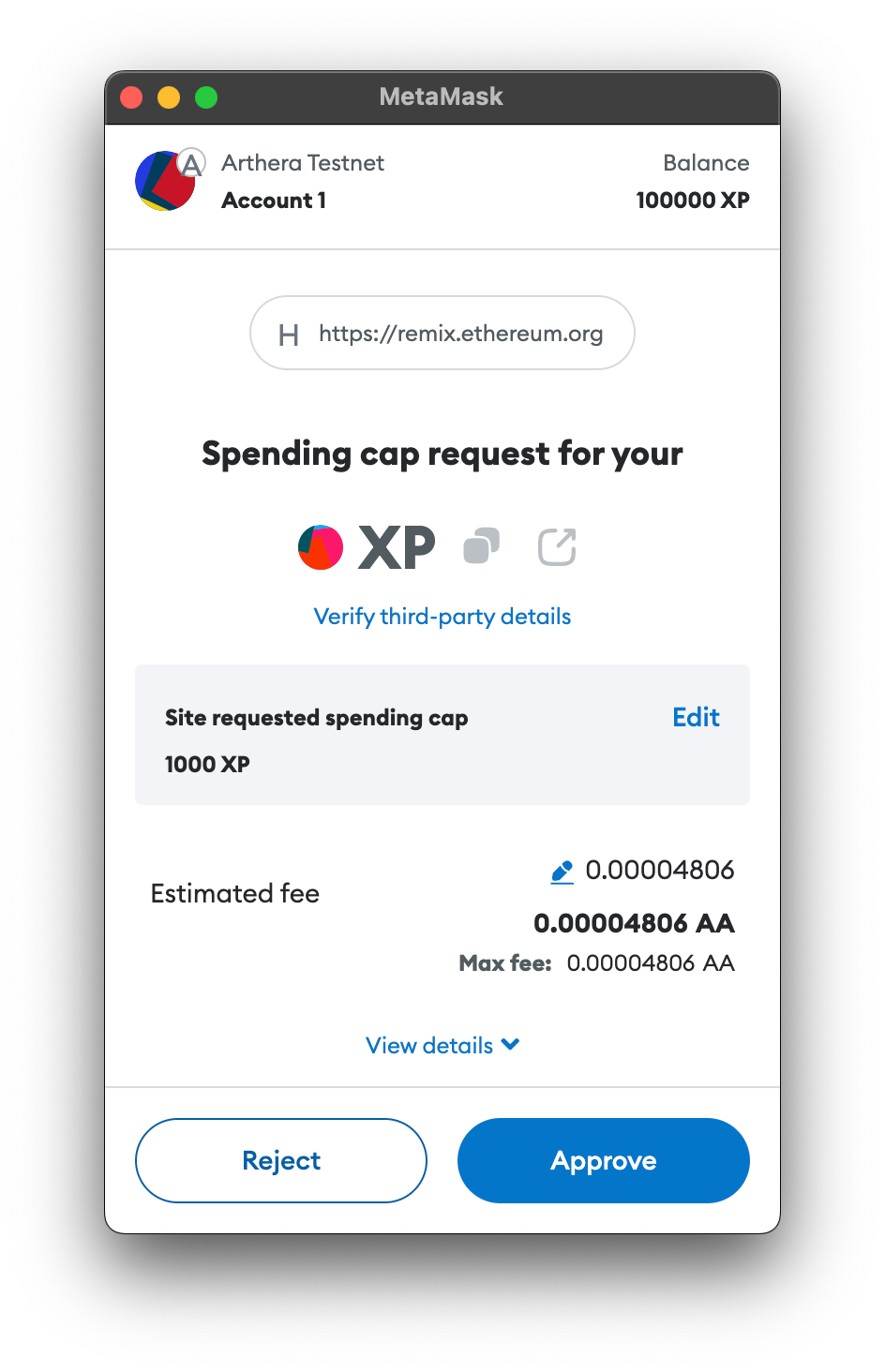
The wallet address used to deploy the contract acts as the token vault and the reward distributor address acts as the distributor of the token.
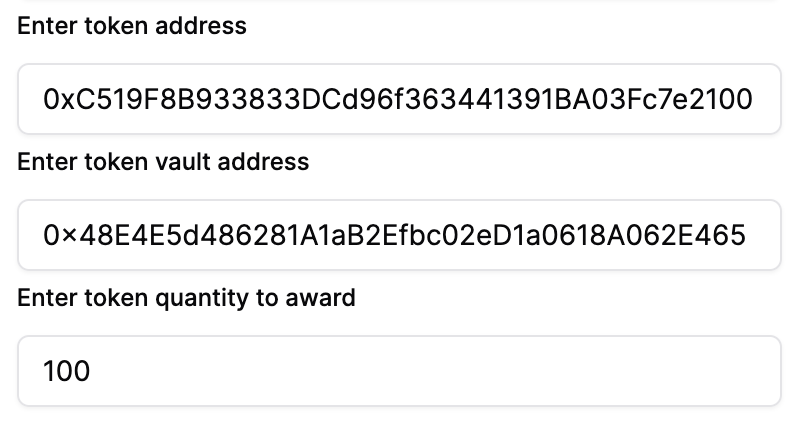
Conclusion
You have successfully deployed an ERC20 token contract using the Remix IDE. You can now use this token in our ERC20 Reward App to incentivize your users to participate in your campaign.